Phase 5: Connect your client applications directly to the target
At this point in our migration phases, we’ve completed:
-
Phase 1: Connected client applications to ZDM Proxy, which included setting up Ansible playbooks with ZDM Utility and using ZDM Proxy Automation to deploy the ZDM Proxy instances with the Docker container.
-
Phase 2: Migrated and validated our data with Cassandra Data Migrator and/or DSBulk Migrator.
-
Phase 3: Optionally enabled async data reads to check that the target cluster can handle the full production workload of read/write traffic.
-
Phase 4: Changed read routing to the target cluster.
In Phase 5 you will configure your client applications to connect directly to the target cluster. How you do this depends on whether your target cluster is Astra DB, Apache Cassandra®, or DSE.
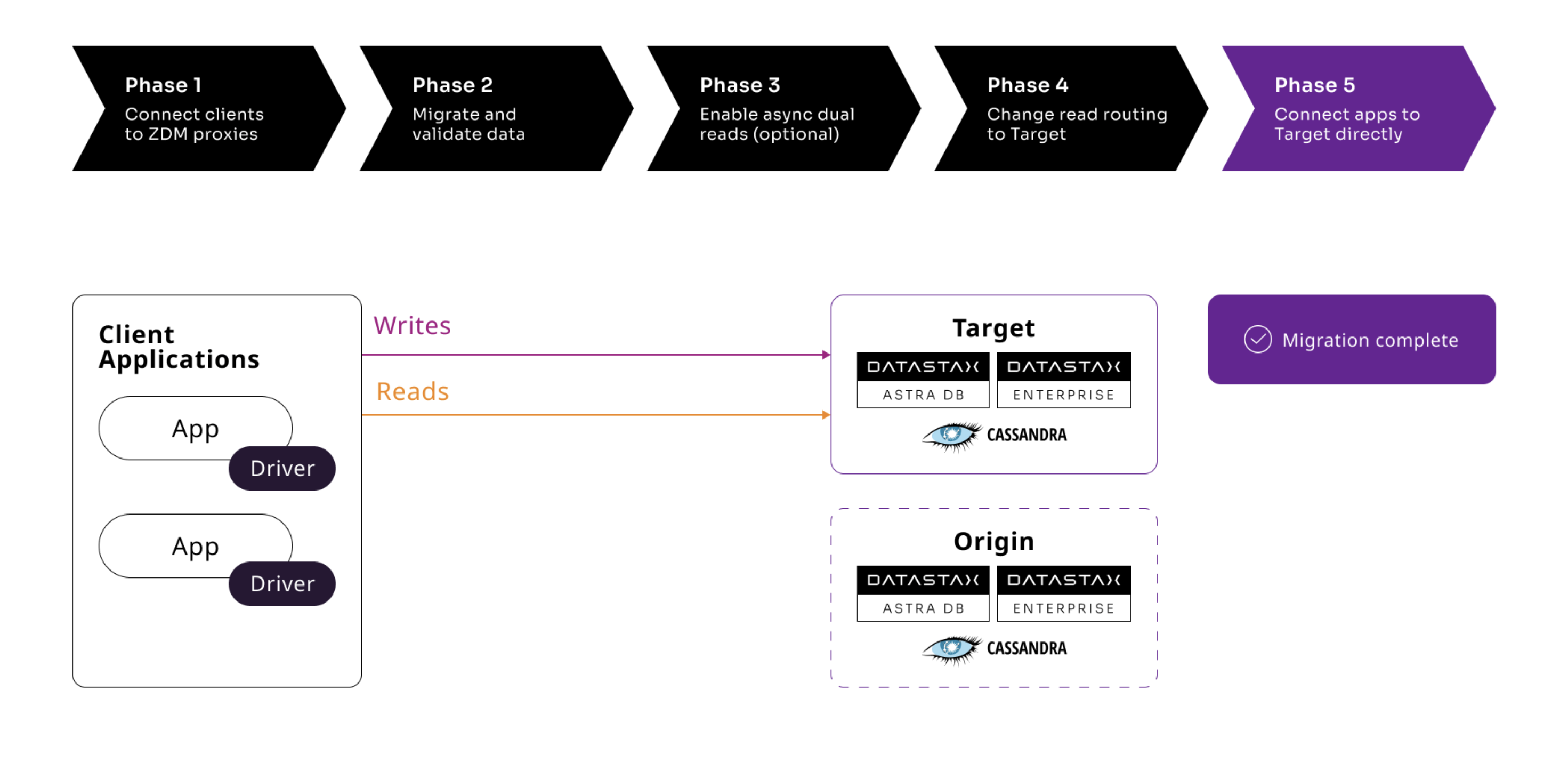
Configuring your driver to connect to a generic CQL cluster
If your target cluster is a generic CQL cluster, such as Cassandra or DSE, then you can connect your client application to it in a similar way as you previously connected it to the origin cluster, but with the appropriate contact points and any additional configuration that your target cluster may require. For connection details, see the documentation for your driver language and version.
Make sure that your driver version is compatible with your target cluster. For more information, see Cassandra drivers supported by DataStax.
Configuring your driver to connect to Astra DB
To connect to Astra DB, you need the following:
-
The application token credentials that you used to connect your applications to ZDM Proxy.
As before, you can use either of the following sets of credentials to connect to your Astra DB database:
-
Token-only authentication: Set
username
to the literal stringtoken
, and setpassword
to your Astra DB application token. -
Client ID and secret authentication (legacy): Set
username
to theclientId
generated with your application token, and then setpassword
to thesecret
generated with your application token.
-
-
Your Astra DB database’s Secure Connect Bundle (SCB).
The SCB is a zip file that contains TLS encryption certificates and other metadata required to connect to your database. Databases can have one or more SCBs. For more information, see Download and use a Secure Connect Bundle (SCB) with Astra DB Serverless.
The SCB contains sensitive information that establishes a connection to your database, including key pairs and certificates. Treat it as you would any other sensitive values, such as passwords or tokens.
-
Recommended: A driver language and version that is compatible with Astra DB. For more information, see Cassandra drivers supported by DataStax.
If your client application uses an old version of a driver without built-in SCB support, DataStax strongly recommends upgrading to a compatible driver to simplify configuration and get the latest features and bug fixes. However, you can still connect to Astra DB for this migration by using CQL Proxy or extracting the SCB archive and using the individual files to enable mTLS in your driver’s configuration.
If your driver has built-in support for the Astra DB SCB, the changes to enable your application to connect to Astra DB are minimal.
The following pseudocode provides guidance on how you might change your driver’s code to connect directly to Astra DB:
// Create an object to represent a Cassandra cluster
// Note: there is no need to specify contact points when connecting to Astra DB.
// All connection information is implicitly passed in the SCB
Cluster my_cluster = Cluster.build_new_cluster(username="my_AstraDB_client_ID", password="my_AstraDB_client_secret", secure_connect_bundle="/path/to/scb.zip")
// Connect our Cluster object to our Cassandra cluster, returning a Session
Session my_session = my_cluster.connect()
// Execute a query, returning a ResultSet
ResultSet my_result_set = my_session.execute("select release_version from system.local")
// Retrieve the "release_version" column from the first row of our result set
String release_version = my_result_set.first_row().get_column("release_version")
// Close our Session and Cluster
my_session.close()
my_cluster.close()
// Display the release version to the user
print(release_version)
As noted before, this pseudocode is just a guideline to illustrate the changes that are needed. For the specific syntax that applies to your driver, see the following documentation:
Your client application is now able to connect directly to your Astra DB database.
Phase 5 of migration completed
Until this point, in case of any issues, you could have abandoned the migration and rolled back to connect directly to the origin cluster at any time. From this point onward, the clusters will diverge, and the target cluster becomes the source of truth for your client applications and data.