Embeddings
This Langflow feature is currently in public preview. Development is ongoing, and the features and functionality are subject to change. Langflow, and the use of such, is subject to the DataStax Preview Terms. |
Embeddings models convert text into numerical vectors. These embeddings capture the semantic meaning of the input text, and allow LLMs to understand context.
Refer to your specific component’s documentation for more information on parameters.
Use an embeddings model component in a flow
In this example of a document ingestion pipeline, the OpenAI embeddings model is connected to a vector database. The component converts the text chunks into vectors and stores them in the vector database. The vectorized data can be used to inform AI workloads like chatbots, similarity searches, and agents.
This embeddings component uses an OpenAI API key for authentication. Refer to your specific embeddings component’s documentation for more information on authentication.
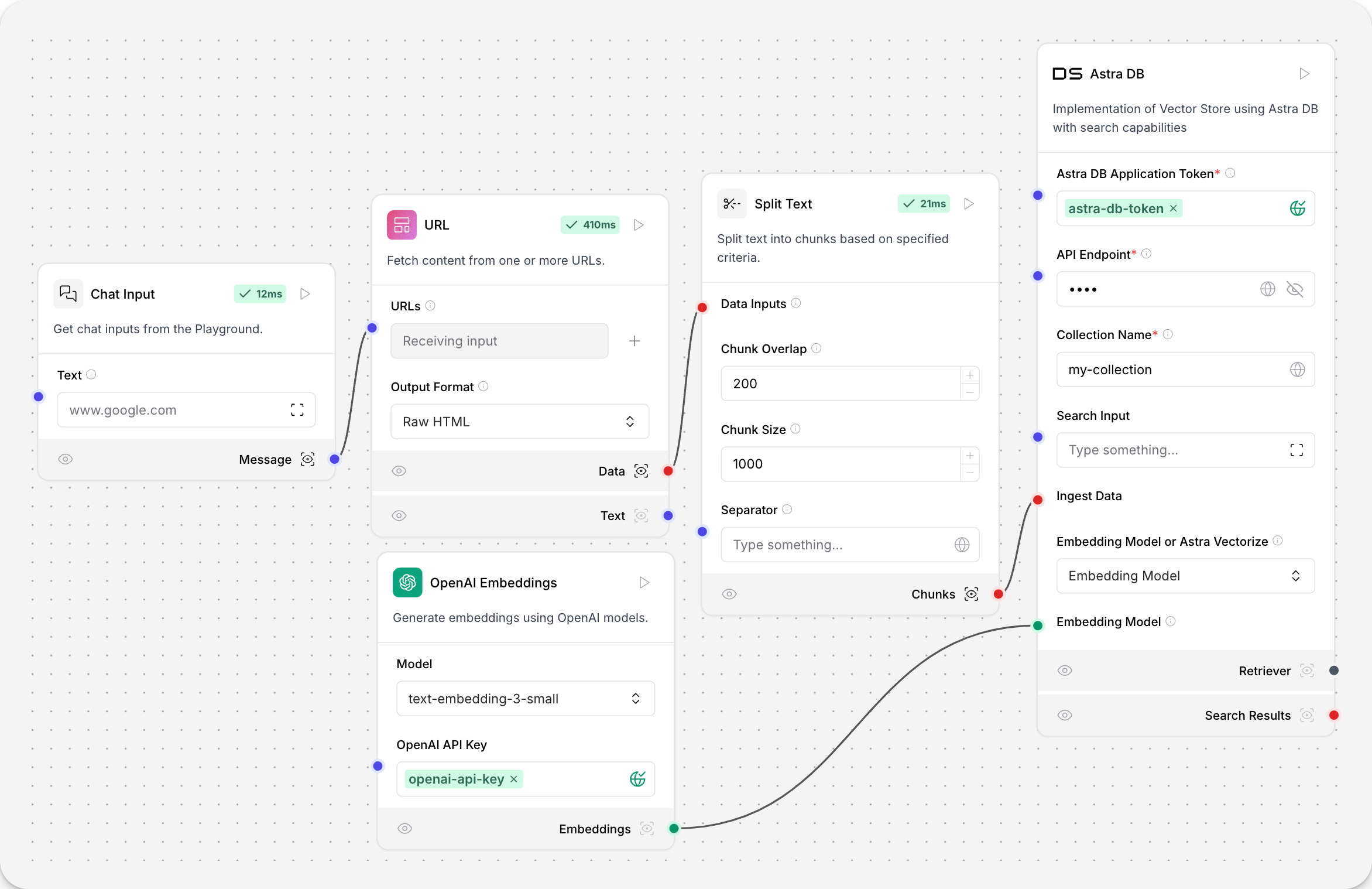
AI/ML
This component generates embeddings using the AI/ML API.
Parameters
Name | Type | Description |
---|---|---|
model_name |
String |
The name of the AI/ML embedding model to use |
aiml_api_key |
SecretString |
API key for authenticating with the AI/ML service |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance of AIMLEmbeddingsImpl for generating embeddings |
Component code
aiml.py
from langflow.base.embeddings.aiml_embeddings import AIMLEmbeddingsImpl
from langflow.base.embeddings.model import LCEmbeddingsModel
from langflow.field_typing import Embeddings
from langflow.inputs.inputs import DropdownInput
from langflow.io import SecretStrInput
class AIMLEmbeddingsComponent(LCEmbeddingsModel):
display_name = "AI/ML Embeddings"
description = "Generate embeddings using the AI/ML API."
icon = "AI/ML"
name = "AIMLEmbeddings"
inputs = [
DropdownInput(
name="model_name",
display_name="Model Name",
options=[
"text-embedding-3-small",
"text-embedding-3-large",
"text-embedding-ada-002",
],
required=True,
),
SecretStrInput(
name="aiml_api_key",
display_name="AI/ML API Key",
value="AIML_API_KEY",
required=True,
),
]
def build_embeddings(self) -> Embeddings:
return AIMLEmbeddingsImpl(
api_key=self.aiml_api_key,
model=self.model_name,
)
Amazon Bedrock Embeddings
Use this component to load embedding models and generate embeddings with Amazon Bedrock.
This component requires an AWS account and access to Amazon Bedrock.
Parameters
Name | Display Name | Info |
---|---|---|
credentials_profile_name |
AWS Credentials Profile |
Name of the AWS credentials profile in ~/.aws/credentials or ~/.aws/config |
model_id |
Model ID |
ID of the model to call, e.g., amazon.titan-embed-text-v1 |
endpoint_url |
Endpoint URL |
URL to set a specific service endpoint other than the default AWS endpoint |
region_name |
AWS Region |
AWS region to use, e.g., us-west-2 |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using Amazon Bedrock. |
Component code
amazon_bedrock.py
404: Not Found
Astra DB vectorize
This component is deprecated as of Langflow version 1.1.2. Instead, use the Astra DB vector store component. |
Connect this component to the Embeddings port of the Astra DB vector store component to generate embeddings.
This component requires that your Astra DB database has a collection that uses a vectorize embedding provider integration. For more information and instructions, see Auto-generate embeddings with vectorize.
Parameters
Name | Display Name | Info |
---|---|---|
provider |
Embedding Provider |
The embedding provider to use |
model_name |
Model Name |
The embedding model to use |
authentication |
Authentication |
The name of the API key in Astra KMS that stores your vectorize embedding provider credentials. (Not required if using an Astra-hosted embedding provider). |
provider_api_key |
Provider API Key |
As an alternative to |
model_parameters |
Model Parameters |
Additional model parameters |
Component code
astra_vectorize.py
from typing import Any
from langflow.custom import Component
from langflow.inputs.inputs import DictInput, DropdownInput, MessageTextInput, SecretStrInput
from langflow.template.field.base import Output
class AstraVectorizeComponent(Component):
display_name: str = "Astra Vectorize [DEPRECATED]"
description: str = (
"Configuration options for Astra Vectorize server-side embeddings. "
"This component is deprecated. Please use the Astra DB Component directly."
)
documentation: str = "https://docs.datastax.com/en/astra-db-serverless/databases/embedding-generation.html"
icon = "AstraDB"
name = "AstraVectorize"
VECTORIZE_PROVIDERS_MAPPING = {
"Azure OpenAI": ["azureOpenAI", ["text-embedding-3-small", "text-embedding-3-large", "text-embedding-ada-002"]],
"Hugging Face - Dedicated": ["huggingfaceDedicated", ["endpoint-defined-model"]],
"Hugging Face - Serverless": [
"huggingface",
[
"sentence-transformers/all-MiniLM-L6-v2",
"intfloat/multilingual-e5-large",
"intfloat/multilingual-e5-large-instruct",
"BAAI/bge-small-en-v1.5",
"BAAI/bge-base-en-v1.5",
"BAAI/bge-large-en-v1.5",
],
],
"Jina AI": [
"jinaAI",
[
"jina-embeddings-v2-base-en",
"jina-embeddings-v2-base-de",
"jina-embeddings-v2-base-es",
"jina-embeddings-v2-base-code",
"jina-embeddings-v2-base-zh",
],
],
"Mistral AI": ["mistral", ["mistral-embed"]],
"NVIDIA": ["nvidia", ["NV-Embed-QA"]],
"OpenAI": ["openai", ["text-embedding-3-small", "text-embedding-3-large", "text-embedding-ada-002"]],
"Upstage": ["upstageAI", ["solar-embedding-1-large"]],
"Voyage AI": [
"voyageAI",
["voyage-large-2-instruct", "voyage-law-2", "voyage-code-2", "voyage-large-2", "voyage-2"],
],
}
VECTORIZE_MODELS_STR = "\n\n".join(
[provider + ": " + (", ".join(models[1])) for provider, models in VECTORIZE_PROVIDERS_MAPPING.items()]
)
inputs = [
DropdownInput(
name="provider",
display_name="Provider",
options=VECTORIZE_PROVIDERS_MAPPING.keys(),
value="",
required=True,
),
MessageTextInput(
name="model_name",
display_name="Model Name",
info="The embedding model to use for the selected provider. Each provider has a different set of models "
f"available (full list at https://docs.datastax.com/en/astra-db-serverless/databases/embedding-generation.html):\n\n{VECTORIZE_MODELS_STR}",
required=True,
),
MessageTextInput(
name="api_key_name",
display_name="API Key name",
info="The name of the embeddings provider API key stored on Astra. "
"If set, it will override the 'ProviderKey' in the authentication parameters.",
),
DictInput(
name="authentication",
display_name="Authentication parameters",
is_list=True,
advanced=True,
),
SecretStrInput(
name="provider_api_key",
display_name="Provider API Key",
info="An alternative to the Astra Authentication that passes an API key for the provider with each request "
"to Astra DB. "
"This may be used when Vectorize is configured for the collection, "
"but no corresponding provider secret is stored within Astra's key management system.",
advanced=True,
),
DictInput(
name="authentication",
display_name="Authentication Parameters",
is_list=True,
advanced=True,
),
DictInput(
name="model_parameters",
display_name="Model Parameters",
advanced=True,
is_list=True,
),
]
outputs = [
Output(display_name="Vectorize", name="config", method="build_options", types=["dict"]),
]
def build_options(self) -> dict[str, Any]:
provider_value = self.VECTORIZE_PROVIDERS_MAPPING[self.provider][0]
authentication = {**(self.authentication or {})}
api_key_name = self.api_key_name
if api_key_name:
authentication["providerKey"] = api_key_name
return {
# must match astrapy.info.VectorServiceOptions
"collection_vector_service_options": {
"provider": provider_value,
"modelName": self.model_name,
"authentication": authentication,
"parameters": self.model_parameters or {},
},
"collection_embedding_api_key": self.provider_api_key,
}
Azure OpenAI Embeddings
This component generates embeddings using Azure OpenAI models.
Use this component to create embeddings with Azure’s OpenAI service.
Make sure you have the necessary Azure credentials and have set up the OpenAI resource.
Parameters
Name | Display Name | Info |
---|---|---|
Azure Endpoint |
Azure Endpoint |
Your Azure endpoint, including the resource |
Deployment Name |
Deployment Name |
The name of the deployment |
API Version |
API Version |
The API version to use |
API Key |
API Key |
The API key to access the Azure OpenAI service |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using Azure OpenAI. |
Component code
azure_openai.py
from langchain_openai import AzureOpenAIEmbeddings
from langflow.base.models.model import LCModelComponent
from langflow.base.models.openai_constants import OPENAI_EMBEDDING_MODEL_NAMES
from langflow.field_typing import Embeddings
from langflow.io import DropdownInput, IntInput, MessageTextInput, Output, SecretStrInput
class AzureOpenAIEmbeddingsComponent(LCModelComponent):
display_name: str = "Azure OpenAI Embeddings"
description: str = "Generate embeddings using Azure OpenAI models."
documentation: str = "https://python.langchain.com/docs/integrations/text_embedding/azureopenai"
icon = "Azure"
name = "AzureOpenAIEmbeddings"
API_VERSION_OPTIONS = [
"2022-12-01",
"2023-03-15-preview",
"2023-05-15",
"2023-06-01-preview",
"2023-07-01-preview",
"2023-08-01-preview",
]
inputs = [
DropdownInput(
name="model",
display_name="Model",
advanced=False,
options=OPENAI_EMBEDDING_MODEL_NAMES,
value=OPENAI_EMBEDDING_MODEL_NAMES[0],
),
MessageTextInput(
name="azure_endpoint",
display_name="Azure Endpoint",
required=True,
info="Your Azure endpoint, including the resource. Example: `https://example-resource.azure.openai.com/`",
),
MessageTextInput(
name="azure_deployment",
display_name="Deployment Name",
required=True,
),
DropdownInput(
name="api_version",
display_name="API Version",
options=API_VERSION_OPTIONS,
value=API_VERSION_OPTIONS[-1],
advanced=True,
),
SecretStrInput(
name="api_key",
display_name="API Key",
required=True,
),
IntInput(
name="dimensions",
display_name="Dimensions",
info="The number of dimensions the resulting output embeddings should have. "
"Only supported by certain models.",
advanced=True,
),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
try:
embeddings = AzureOpenAIEmbeddings(
model=self.model,
azure_endpoint=self.azure_endpoint,
azure_deployment=self.azure_deployment,
api_version=self.api_version,
api_key=self.api_key,
dimensions=self.dimensions or None,
)
except Exception as e:
msg = f"Could not connect to AzureOpenAIEmbeddings API: {e}"
raise ValueError(msg) from e
return embeddings
Cloudflare Workers AI Embeddings
This component generates embeddings using Cloudflare Workers AI models.
Parameters
Name | Display Name | Info |
---|---|---|
account_id |
Cloudflare account ID |
|
api_token |
Cloudflare API token |
|
model_name |
Model Name |
|
strip_new_lines |
Strip New Lines |
Whether to strip new lines from the input text. |
batch_size |
Batch Size |
Number of texts to embed in each batch. |
api_base_url |
Cloudflare API base URL |
Base URL for the Cloudflare API. |
headers |
Headers |
Additional request headers. |
Name | Display Name | Info |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using Cloudflare Workers. |
Component code
cloudflare.py
from langchain_community.embeddings.cloudflare_workersai import CloudflareWorkersAIEmbeddings
from langflow.base.models.model import LCModelComponent
from langflow.field_typing import Embeddings
from langflow.io import BoolInput, DictInput, IntInput, MessageTextInput, Output, SecretStrInput
class CloudflareWorkersAIEmbeddingsComponent(LCModelComponent):
display_name: str = "Cloudflare Workers AI Embeddings"
description: str = "Generate embeddings using Cloudflare Workers AI models."
documentation: str = "https://python.langchain.com/docs/integrations/text_embedding/cloudflare_workersai/"
icon = "Cloudflare"
name = "CloudflareWorkersAIEmbeddings"
inputs = [
MessageTextInput(
name="account_id",
display_name="Cloudflare account ID",
info="Find your account ID https://developers.cloudflare.com/fundamentals/setup/find-account-and-zone-ids/#find-account-id-workers-and-pages",
required=True,
),
SecretStrInput(
name="api_token",
display_name="Cloudflare API token",
info="Create an API token https://developers.cloudflare.com/fundamentals/api/get-started/create-token/",
required=True,
),
MessageTextInput(
name="model_name",
display_name="Model Name",
info="List of supported models https://developers.cloudflare.com/workers-ai/models/#text-embeddings",
required=True,
value="@cf/baai/bge-base-en-v1.5",
),
BoolInput(
name="strip_new_lines",
display_name="Strip New Lines",
advanced=True,
value=True,
),
IntInput(
name="batch_size",
display_name="Batch Size",
advanced=True,
value=50,
),
MessageTextInput(
name="api_base_url",
display_name="Cloudflare API base URL",
advanced=True,
value="https://api.cloudflare.com/client/v4/accounts",
),
DictInput(
name="headers",
display_name="Headers",
info="Additional request headers",
is_list=True,
advanced=True,
),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
try:
embeddings = CloudflareWorkersAIEmbeddings(
account_id=self.account_id,
api_base_url=self.api_base_url,
api_token=self.api_token,
batch_size=self.batch_size,
headers=self.headers,
model_name=self.model_name,
strip_new_lines=self.strip_new_lines,
)
except Exception as e:
msg = f"Could not connect to CloudflareWorkersAIEmbeddings API: {e!s}"
raise ValueError(msg) from e
return embeddings
Cohere Embeddings
This component loads embedding models from Cohere.
Use this component to generate embeddings using Cohere’s AI models.
Ensure you have a valid Cohere API key.
Parameters
Name | Display Name | Info |
---|---|---|
cohere_api_key |
Cohere API Key |
API key required to authenticate with the Cohere service. |
model |
Model Name |
Language model used for embedding text documents and performing queries. |
truncate |
Truncate |
Whether to truncate the input text to fit within the model’s constraints. |
Name | Display Name | Info |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using Cohere. |
Component code
cohere.py
from typing import Any
import cohere
from langchain_cohere import CohereEmbeddings
from langflow.base.models.model import LCModelComponent
from langflow.field_typing import Embeddings
from langflow.io import DropdownInput, FloatInput, IntInput, MessageTextInput, Output, SecretStrInput
HTTP_STATUS_OK = 200
class CohereEmbeddingsComponent(LCModelComponent):
display_name = "Cohere Embeddings"
description = "Generate embeddings using Cohere models."
icon = "Cohere"
name = "CohereEmbeddings"
inputs = [
SecretStrInput(name="api_key", display_name="Cohere API Key", required=True, real_time_refresh=True),
DropdownInput(
name="model_name",
display_name="Model",
advanced=False,
options=[
"embed-english-v2.0",
"embed-multilingual-v2.0",
"embed-english-light-v2.0",
"embed-multilingual-light-v2.0",
],
value="embed-english-v2.0",
refresh_button=True,
combobox=True,
),
MessageTextInput(name="truncate", display_name="Truncate", advanced=True),
IntInput(name="max_retries", display_name="Max Retries", value=3, advanced=True),
MessageTextInput(name="user_agent", display_name="User Agent", advanced=True, value="langchain"),
FloatInput(name="request_timeout", display_name="Request Timeout", advanced=True),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
data = None
try:
data = CohereEmbeddings(
cohere_api_key=self.api_key,
model=self.model_name,
truncate=self.truncate,
max_retries=self.max_retries,
user_agent=self.user_agent,
request_timeout=self.request_timeout or None,
)
except Exception as e:
msg = (
"Unable to create Cohere Embeddings. ",
"Please verify the API key and model parameters, and try again.",
)
raise ValueError(msg) from e
# added status if not the return data would be serialised to create the status
return data
def get_model(self):
try:
co = cohere.ClientV2(self.api_key)
response = co.models.list(endpoint="embed")
models = response.models
return [model.name for model in models]
except Exception as e:
msg = f"Failed to fetch Cohere models. Error: {e}"
raise ValueError(msg) from e
async def update_build_config(self, build_config: dict, field_value: Any, field_name: str | None = None):
if field_name in {"model_name", "api_key"}:
if build_config.get("api_key", {}).get("value", None):
build_config["model_name"]["options"] = self.get_model()
else:
build_config["model_name"]["options"] = field_value
return build_config
Embedding similarity
This component computes selected forms of similarity between two embedding vectors.
Parameters
Name | Display Name | Info |
---|---|---|
embedding_vectors |
Embedding Vectors |
A list containing exactly two data objects with embedding vectors to compare. |
similarity_metric |
Similarity Metric |
Select the similarity metric to use. Options: "Cosine Similarity", "Euclidean Distance", "Manhattan Distance". |
Name | Display Name | Info |
---|---|---|
similarity_data |
Similarity Data |
Data object containing the computed similarity score and additional information. |
Component code
similarity.py
import numpy as np
from langflow.custom import Component
from langflow.io import DataInput, DropdownInput, Output
from langflow.schema import Data
class EmbeddingSimilarityComponent(Component):
display_name: str = "Embedding Similarity"
description: str = "Compute selected form of similarity between two embedding vectors."
icon = "equal"
inputs = [
DataInput(
name="embedding_vectors",
display_name="Embedding Vectors",
info="A list containing exactly two data objects with embedding vectors to compare.",
is_list=True,
required=True,
),
DropdownInput(
name="similarity_metric",
display_name="Similarity Metric",
info="Select the similarity metric to use.",
options=["Cosine Similarity", "Euclidean Distance", "Manhattan Distance"],
value="Cosine Similarity",
),
]
outputs = [
Output(display_name="Similarity Data", name="similarity_data", method="compute_similarity"),
]
def compute_similarity(self) -> Data:
embedding_vectors: list[Data] = self.embedding_vectors
# Assert that the list contains exactly two Data objects
if len(embedding_vectors) != 2: # noqa: PLR2004
msg = "Exactly two embedding vectors are required."
raise ValueError(msg)
embedding_1 = np.array(embedding_vectors[0].data["embeddings"])
embedding_2 = np.array(embedding_vectors[1].data["embeddings"])
if embedding_1.shape != embedding_2.shape:
similarity_score = {"error": "Embeddings must have the same dimensions."}
else:
similarity_metric = self.similarity_metric
if similarity_metric == "Cosine Similarity":
score = np.dot(embedding_1, embedding_2) / (np.linalg.norm(embedding_1) * np.linalg.norm(embedding_2))
similarity_score = {"cosine_similarity": score}
elif similarity_metric == "Euclidean Distance":
score = np.linalg.norm(embedding_1 - embedding_2)
similarity_score = {"euclidean_distance": score}
elif similarity_metric == "Manhattan Distance":
score = np.sum(np.abs(embedding_1 - embedding_2))
similarity_score = {"manhattan_distance": score}
# Create a Data object to encapsulate the similarity score and additional information
similarity_data = Data(
data={
"embedding_1": embedding_vectors[0].data["embeddings"],
"embedding_2": embedding_vectors[1].data["embeddings"],
"similarity_score": similarity_score,
},
text_key="similarity_score",
)
self.status = similarity_data
return similarity_data
Google generative AI embeddings
This component connects to Google’s generative AI embedding service using the GoogleGenerativeAIEmbeddings class from the langchain-google-genai
package.
Parameters
Name | Display Name | Info |
---|---|---|
api_key |
API Key |
Secret API key for accessing Google’s generative AI service (required) |
model_name |
Model Name |
Name of the embedding model to use (default: "models/text-embedding-004") |
Name | Display Name | Info |
---|---|---|
embeddings |
Embeddings |
Built GoogleGenerativeAIEmbeddings object. |
Component code
google_generative_ai.py
# from langflow.field_typing import Data
# TODO: remove ignore once the google package is published with types
from google.ai.generativelanguage_v1beta.types import BatchEmbedContentsRequest
from langchain_core.embeddings import Embeddings
from langchain_google_genai import GoogleGenerativeAIEmbeddings
from langchain_google_genai._common import GoogleGenerativeAIError
from langflow.custom import Component
from langflow.io import MessageTextInput, Output, SecretStrInput
MIN_DIMENSION_ERROR = "Output dimensionality must be at least 1"
MAX_DIMENSION_ERROR = (
"Output dimensionality cannot exceed 768. Google's embedding models only support dimensions up to 768."
)
MAX_DIMENSION = 768
MIN_DIMENSION = 1
class GoogleGenerativeAIEmbeddingsComponent(Component):
display_name = "Google Generative AI Embeddings"
description = (
"Connect to Google's generative AI embeddings service using the GoogleGenerativeAIEmbeddings class, "
"found in the langchain-google-genai package."
)
documentation: str = "https://python.langchain.com/v0.2/docs/integrations/text_embedding/google_generative_ai/"
icon = "Google"
name = "Google Generative AI Embeddings"
inputs = [
SecretStrInput(name="api_key", display_name="API Key", required=True),
MessageTextInput(name="model_name", display_name="Model Name", value="models/text-embedding-004"),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
if not self.api_key:
msg = "API Key is required"
raise ValueError(msg)
class HotaGoogleGenerativeAIEmbeddings(GoogleGenerativeAIEmbeddings):
def __init__(self, *args, **kwargs) -> None:
super(GoogleGenerativeAIEmbeddings, self).__init__(*args, **kwargs)
def embed_documents(
self,
texts: list[str],
*,
batch_size: int = 100,
task_type: str | None = None,
titles: list[str] | None = None,
output_dimensionality: int | None = 768,
) -> list[list[float]]:
"""Embed a list of strings.
Google Generative AI currently sets a max batch size of 100 strings.
Args:
texts: List[str] The list of strings to embed.
batch_size: [int] The batch size of embeddings to send to the model
task_type: task_type (https://ai.google.dev/api/rest/v1/TaskType)
titles: An optional list of titles for texts provided.
Only applicable when TaskType is RETRIEVAL_DOCUMENT.
output_dimensionality: Optional reduced dimension for the output embedding.
https://ai.google.dev/api/rest/v1/models/batchEmbedContents#EmbedContentRequest
Returns:
List of embeddings, one for each text.
"""
if output_dimensionality is not None and output_dimensionality < MIN_DIMENSION:
raise ValueError(MIN_DIMENSION_ERROR)
if output_dimensionality is not None and output_dimensionality > MAX_DIMENSION:
error_msg = MAX_DIMENSION_ERROR.format(output_dimensionality)
raise ValueError(error_msg)
embeddings: list[list[float]] = []
batch_start_index = 0
for batch in GoogleGenerativeAIEmbeddings._prepare_batches(texts, batch_size):
if titles:
titles_batch = titles[batch_start_index : batch_start_index + len(batch)]
batch_start_index += len(batch)
else:
titles_batch = [None] * len(batch) # type: ignore[list-item]
requests = [
self._prepare_request(
text=text,
task_type=task_type,
title=title,
output_dimensionality=output_dimensionality,
)
for text, title in zip(batch, titles_batch, strict=True)
]
try:
result = self.client.batch_embed_contents(
BatchEmbedContentsRequest(requests=requests, model=self.model)
)
except Exception as e:
msg = f"Error embedding content: {e}"
raise GoogleGenerativeAIError(msg) from e
embeddings.extend([list(e.values) for e in result.embeddings])
return embeddings
def embed_query(
self,
text: str,
task_type: str | None = None,
title: str | None = None,
output_dimensionality: int | None = 768,
) -> list[float]:
"""Embed a text.
Args:
text: The text to embed.
task_type: task_type (https://ai.google.dev/api/rest/v1/TaskType)
title: An optional title for the text.
Only applicable when TaskType is RETRIEVAL_DOCUMENT.
output_dimensionality: Optional reduced dimension for the output embedding.
https://ai.google.dev/api/rest/v1/models/batchEmbedContents#EmbedContentRequest
Returns:
Embedding for the text.
"""
if output_dimensionality is not None and output_dimensionality < MIN_DIMENSION:
raise ValueError(MIN_DIMENSION_ERROR)
if output_dimensionality is not None and output_dimensionality > MAX_DIMENSION:
error_msg = MAX_DIMENSION_ERROR.format(output_dimensionality)
raise ValueError(error_msg)
task_type = task_type or "RETRIEVAL_QUERY"
return self.embed_documents(
[text],
task_type=task_type,
titles=[title] if title else None,
output_dimensionality=output_dimensionality,
)[0]
return HotaGoogleGenerativeAIEmbeddings(model=self.model_name, google_api_key=self.api_key)
Hugging Face Embeddings
This component is deprecated as of Langflow version 1.0.18. Instead, use the Hugging Face API Embeddings component. |
This component loads embedding models from HuggingFace.
Use this component to generate embeddings using locally downloaded Hugging Face models. Ensure you have sufficient computational resources to run the models.
Parameters
Name | Display Name | Info |
---|---|---|
Cache Folder |
Cache Folder |
Folder path to cache HuggingFace models |
Encode Kwargs |
Encoding Arguments |
Additional arguments for the encoding process |
Model Kwargs |
Model Arguments |
Additional arguments for the model |
Model Name |
Model Name |
Name of the HuggingFace model to use |
Multi Process |
Multi-Process |
Whether to use multiple processes |
Hugging Face embeddings inference
This component generates embeddings using Hugging Face inference API models and requires a Hugging Face API token to authenticate. Local inference models do not require an API key.
Use this component to create embeddings with Hugging Face’s hosted models, or to connect to your own locally hosted models. This component generates embeddings using Inference API models.
Use this component to create embeddings with Hugging Face’s hosted models. Ensure you have a valid Hugging Face API key.
Parameters
Name | Display name | Description |
---|---|---|
API Key |
API Key |
The API key for accessing the Hugging Face Inference API. |
API URL |
API URL |
The URL of the Hugging Face Inference API. |
Model Name |
Model Name |
The name of the model to use for embeddings. |
Cache Folder |
Cache Folder |
The folder path to cache Hugging Face models. |
Encode Kwargs |
Encoding Arguments |
Additional arguments for the encoding process. |
Model Kwargs |
Model Arguments |
Additional arguments for the model. |
Multi Process |
Multi-Process |
Whether to use multiple processes. |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using HuggingFace. |
Component code
huggingface_inference_api.py
from urllib.parse import urlparse
import requests
from langchain_community.embeddings.huggingface import HuggingFaceInferenceAPIEmbeddings
# Next update: use langchain_huggingface
from pydantic import SecretStr
from tenacity import retry, stop_after_attempt, wait_fixed
from langflow.base.embeddings.model import LCEmbeddingsModel
from langflow.field_typing import Embeddings
from langflow.io import MessageTextInput, Output, SecretStrInput
class HuggingFaceInferenceAPIEmbeddingsComponent(LCEmbeddingsModel):
display_name = "HuggingFace Embeddings Inference"
description = "Generate embeddings using HuggingFace Text Embeddings Inference (TEI)"
documentation = "https://huggingface.co/docs/text-embeddings-inference/index"
icon = "HuggingFace"
name = "HuggingFaceInferenceAPIEmbeddings"
inputs = [
SecretStrInput(
name="api_key",
display_name="API Key",
advanced=False,
info="Required for non-local inference endpoints. Local inference does not require an API Key.",
),
MessageTextInput(
name="inference_endpoint",
display_name="Inference Endpoint",
required=True,
value="https://api-inference.huggingface.co/models/",
info="Custom inference endpoint URL.",
),
MessageTextInput(
name="model_name",
display_name="Model Name",
value="BAAI/bge-large-en-v1.5",
info="The name of the model to use for text embeddings.",
required=True,
),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def validate_inference_endpoint(self, inference_endpoint: str) -> bool:
parsed_url = urlparse(inference_endpoint)
if not all([parsed_url.scheme, parsed_url.netloc]):
msg = (
f"Invalid inference endpoint format: '{self.inference_endpoint}'. "
"Please ensure the URL includes both a scheme (e.g., 'http://' or 'https://') and a domain name. "
"Example: 'http://localhost:8080' or 'https://api.example.com'"
)
raise ValueError(msg)
try:
response = requests.get(f"{inference_endpoint}/health", timeout=5)
except requests.RequestException as e:
msg = (
f"Inference endpoint '{inference_endpoint}' is not responding. "
"Please ensure the URL is correct and the service is running."
)
raise ValueError(msg) from e
if response.status_code != requests.codes.ok:
msg = f"HuggingFace health check failed: {response.status_code}"
raise ValueError(msg)
# returning True to solve linting error
return True
def get_api_url(self) -> str:
if "huggingface" in self.inference_endpoint.lower():
return f"{self.inference_endpoint}"
return self.inference_endpoint
@retry(stop=stop_after_attempt(3), wait=wait_fixed(2))
def create_huggingface_embeddings(
self, api_key: SecretStr, api_url: str, model_name: str
) -> HuggingFaceInferenceAPIEmbeddings:
return HuggingFaceInferenceAPIEmbeddings(api_key=api_key, api_url=api_url, model_name=model_name)
def build_embeddings(self) -> Embeddings:
api_url = self.get_api_url()
is_local_url = (
api_url.startswith(("http://localhost", "http://127.0.0.1", "http://0.0.0.0", "http://docker"))
or "huggingface.co" not in api_url.lower()
)
if not self.api_key and is_local_url:
self.validate_inference_endpoint(api_url)
api_key = SecretStr("APIKeyForLocalDeployment")
elif not self.api_key:
msg = "API Key is required for non-local inference endpoints"
raise ValueError(msg)
else:
api_key = SecretStr(self.api_key).get_secret_value()
try:
return self.create_huggingface_embeddings(api_key, api_url, self.model_name)
except Exception as e:
msg = "Could not connect to HuggingFace Inference API."
raise ValueError(msg) from e
Connect the Hugging Face component to a local embeddings model
To run an embeddings inference model locally, see the Hugging Face documentation.
To connect the local Hugging Face model to the Hugging Face embeddings inference component and use it in a flow, follow these steps:
-
Create a Vector store RAG flow. There are two embeddings models in this flow that you can replace with Hugging Face embeddings inference components.
-
Replace both OpenAI embeddings model components with Hugging Face model components.
-
Connect both Hugging Face components to the Embeddings ports of the Astra DB vector store components.
-
In the Hugging Face components, set the Inference Endpoint field to the URL of your local inference model. The API Key field is not required for local inference.
-
Run the flow. The local inference models generate embeddings for the input text.
IBM watsonx.ai Embeddings
This component generates embeddings using IBM watsonx.aimodels.
Parameters
Name | Display Name | Info |
---|---|---|
url |
watsonx API Endpoint |
The base URL of the watsonx API. |
project_id |
watsonx project id |
The project ID for watsonx.ai. |
api_key |
API Key |
The API Key to use for the model. |
model_name |
Model Name |
The name of the watsonx embeddings model to use. Options are dynamically fetched from the API. |
truncate_input_tokens |
Truncate Input Tokens |
Maximum number of input tokens. The default is 200 tokens. |
input_text |
Include the original text in the output |
Whether to include the original text in the output. The default is |
Name | Display Name | Info |
---|---|---|
embeddings |
Embeddings |
The configured watsonx.ai embeddings model. Type: Embeddings |
Component code
watsonx.py
from typing import Any
import requests
from ibm_watsonx_ai import APIClient, Credentials
from ibm_watsonx_ai.metanames import EmbedTextParamsMetaNames
from langchain_ibm import WatsonxEmbeddings
from loguru import logger
from pydantic.v1 import SecretStr
from langflow.base.embeddings.model import LCEmbeddingsModel
from langflow.field_typing import Embeddings
from langflow.io import BoolInput, DropdownInput, IntInput, SecretStrInput, StrInput
from langflow.schema.dotdict import dotdict
class WatsonxEmbeddingsComponent(LCEmbeddingsModel):
display_name = "IBM watsonx.ai Embeddings"
description = "Generate embeddings using IBM watsonx.ai models."
icon = "WatsonxAI"
name = "WatsonxEmbeddingsComponent"
# models present in all the regions
_default_models = [
"sentence-transformers/all-minilm-l12-v2",
"ibm/slate-125m-english-rtrvr-v2",
"ibm/slate-30m-english-rtrvr-v2",
"intfloat/multilingual-e5-large",
]
inputs = [
DropdownInput(
name="url",
display_name="watsonx API Endpoint",
info="The base URL of the API.",
value=None,
options=[
"https://us-south.ml.cloud.ibm.com",
"https://eu-de.ml.cloud.ibm.com",
"https://eu-gb.ml.cloud.ibm.com",
"https://au-syd.ml.cloud.ibm.com",
"https://jp-tok.ml.cloud.ibm.com",
"https://ca-tor.ml.cloud.ibm.com",
],
real_time_refresh=True,
),
StrInput(
name="project_id",
display_name="watsonx project id",
info="The project ID or deployment space ID that is associated with the foundation model.",
required=True,
),
SecretStrInput(
name="api_key",
display_name="API Key",
info="The API Key to use for the model.",
required=True,
),
DropdownInput(
name="model_name",
display_name="Model Name",
options=[],
value=None,
dynamic=True,
required=True,
),
IntInput(
name="truncate_input_tokens",
display_name="Truncate Input Tokens",
advanced=True,
value=200,
),
BoolInput(
name="input_text",
display_name="Include the original text in the output",
value=True,
advanced=True,
),
]
@staticmethod
def fetch_models(base_url: str) -> list[str]:
"""Fetch available models from the watsonx.ai API."""
try:
endpoint = f"{base_url}/ml/v1/foundation_model_specs"
params = {
"version": "2024-09-16",
"filters": "function_embedding,!lifecycle_withdrawn:and",
}
response = requests.get(endpoint, params=params, timeout=10)
response.raise_for_status()
data = response.json()
models = [model["model_id"] for model in data.get("resources", [])]
return sorted(models)
except Exception: # noqa: BLE001
logger.exception("Error fetching models")
return WatsonxEmbeddingsComponent._default_models
def update_build_config(self, build_config: dotdict, field_value: Any, field_name: str | None = None):
"""Update model options when URL or API key changes."""
logger.debug(
"Updating build config. Field name: %s, Field value: %s",
field_name,
field_value,
)
if field_name == "url" and field_value:
try:
models = self.fetch_models(base_url=build_config.url.value)
build_config.model_name.options = models
if build_config.model_name.value:
build_config.model_name.value = models[0]
info_message = f"Updated model options: {len(models)} models found in {build_config.url.value}"
logger.info(info_message)
except Exception: # noqa: BLE001
logger.exception("Error updating model options.")
def build_embeddings(self) -> Embeddings:
credentials = Credentials(
api_key=SecretStr(self.api_key).get_secret_value(),
url=self.url,
)
api_client = APIClient(credentials)
params = {
EmbedTextParamsMetaNames.TRUNCATE_INPUT_TOKENS: self.truncate_input_tokens,
EmbedTextParamsMetaNames.RETURN_OPTIONS: {"input_text": self.input_text},
}
return WatsonxEmbeddings(
model_id=self.model_name,
params=params,
watsonx_client=api_client,
project_id=self.project_id,
)
LM Studio Embeddings
This component generates embeddings using LM Studio models.
Parameters
Name | Display Name | Info |
---|---|---|
model |
Model |
The LM Studio model to use for generating embeddings |
base_url |
LM Studio Base URL |
The base URL for the LM Studio API |
api_key |
LM Studio API Key |
API key for authentication with LM Studio |
temperature |
Model Temperature |
Temperature setting for the model |
Name | Display Name | Info |
---|---|---|
embeddings |
Embeddings |
The generated embeddings. |
Component code
lmstudioembeddings.py
from typing import Any
from urllib.parse import urljoin
import httpx
from typing_extensions import override
from langflow.base.embeddings.model import LCEmbeddingsModel
from langflow.field_typing import Embeddings
from langflow.inputs.inputs import DropdownInput, SecretStrInput
from langflow.io import FloatInput, MessageTextInput
class LMStudioEmbeddingsComponent(LCEmbeddingsModel):
display_name: str = "LM Studio Embeddings"
description: str = "Generate embeddings using LM Studio."
icon = "LMStudio"
@override
async def update_build_config(self, build_config: dict, field_value: Any, field_name: str | None = None):
if field_name == "model":
base_url_dict = build_config.get("base_url", {})
base_url_load_from_db = base_url_dict.get("load_from_db", False)
base_url_value = base_url_dict.get("value")
if base_url_load_from_db:
base_url_value = await self.get_variables(base_url_value, field_name)
elif not base_url_value:
base_url_value = "http://localhost:1234/v1"
build_config["model"]["options"] = await self.get_model(base_url_value)
return build_config
@staticmethod
async def get_model(base_url_value: str) -> list[str]:
try:
url = urljoin(base_url_value, "/v1/models")
async with httpx.AsyncClient() as client:
response = await client.get(url)
response.raise_for_status()
data = response.json()
return [model["id"] for model in data.get("data", [])]
except Exception as e:
msg = "Could not retrieve models. Please, make sure the LM Studio server is running."
raise ValueError(msg) from e
inputs = [
DropdownInput(
name="model",
display_name="Model",
advanced=False,
refresh_button=True,
required=True,
),
MessageTextInput(
name="base_url",
display_name="LM Studio Base URL",
refresh_button=True,
value="http://localhost:1234/v1",
required=True,
),
SecretStrInput(
name="api_key",
display_name="LM Studio API Key",
advanced=True,
value="LMSTUDIO_API_KEY",
),
FloatInput(
name="temperature",
display_name="Model Temperature",
value=0.1,
advanced=True,
),
]
def build_embeddings(self) -> Embeddings:
try:
from langchain_nvidia_ai_endpoints import NVIDIAEmbeddings
except ImportError as e:
msg = "Please install langchain-nvidia-ai-endpoints to use LM Studio Embeddings."
raise ImportError(msg) from e
try:
output = NVIDIAEmbeddings(
model=self.model,
base_url=self.base_url,
temperature=self.temperature,
nvidia_api_key=self.api_key,
)
except Exception as e:
msg = f"Could not connect to LM Studio API. Error: {e}"
raise ValueError(msg) from e
return output
MistralAI Embeddings
This component generates embeddings using MistralAI models.
Parameters
Name | Type | Description |
---|---|---|
model |
String |
The MistralAI model to use (default: "mistral-embed") |
mistral_api_key |
SecretString |
API key for authenticating with MistralAI |
max_concurrent_requests |
Integer |
Maximum number of concurrent API requests (default: 64) |
max_retries |
Integer |
Maximum number of retry attempts for failed requests (default: 5) |
timeout |
Integer |
Request timeout in seconds (default: 120) |
endpoint |
String |
Custom API endpoint URL (default: "https://api.mistral.ai/v1/") |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
MistralAIEmbeddings instance for generating embeddings. |
Component code
mistral.py
from langchain_mistralai.embeddings import MistralAIEmbeddings
from pydantic.v1 import SecretStr
from langflow.base.models.model import LCModelComponent
from langflow.field_typing import Embeddings
from langflow.io import DropdownInput, IntInput, MessageTextInput, Output, SecretStrInput
class MistralAIEmbeddingsComponent(LCModelComponent):
display_name = "MistralAI Embeddings"
description = "Generate embeddings using MistralAI models."
icon = "MistralAI"
name = "MistalAIEmbeddings"
inputs = [
DropdownInput(
name="model",
display_name="Model",
advanced=False,
options=["mistral-embed"],
value="mistral-embed",
),
SecretStrInput(name="mistral_api_key", display_name="Mistral API Key", required=True),
IntInput(
name="max_concurrent_requests",
display_name="Max Concurrent Requests",
advanced=True,
value=64,
),
IntInput(name="max_retries", display_name="Max Retries", advanced=True, value=5),
IntInput(name="timeout", display_name="Request Timeout", advanced=True, value=120),
MessageTextInput(
name="endpoint",
display_name="API Endpoint",
advanced=True,
value="https://api.mistral.ai/v1/",
),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
if not self.mistral_api_key:
msg = "Mistral API Key is required"
raise ValueError(msg)
api_key = SecretStr(self.mistral_api_key).get_secret_value()
return MistralAIEmbeddings(
api_key=api_key,
model=self.model,
endpoint=self.endpoint,
max_concurrent_requests=self.max_concurrent_requests,
max_retries=self.max_retries,
timeout=self.timeout,
)
NVIDIA
This component generates embeddings using NVIDIA models.
Parameters
Name | Type | Description |
---|---|---|
model |
String |
The NVIDIA model to use for embeddings (e.g., |
base_url |
String |
Base URL for the NVIDIA API (default: |
nvidia_api_key |
SecretString |
API key for authenticating with NVIDIA’s service |
temperature |
Float |
Model temperature for embedding generation (default: 0.1) |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
NVIDIAEmbeddings instance for generating embeddings |
Component code
nvidia.py
from typing import Any
from langflow.base.embeddings.model import LCEmbeddingsModel
from langflow.field_typing import Embeddings
from langflow.inputs.inputs import DropdownInput, SecretStrInput
from langflow.io import FloatInput, MessageTextInput
from langflow.schema.dotdict import dotdict
class NVIDIAEmbeddingsComponent(LCEmbeddingsModel):
display_name: str = "NVIDIA Embeddings"
description: str = "Generate embeddings using NVIDIA models."
icon = "NVIDIA"
inputs = [
DropdownInput(
name="model",
display_name="Model",
options=[
"nvidia/nv-embed-v1",
"snowflake/arctic-embed-I",
],
value="nvidia/nv-embed-v1",
required=True,
),
MessageTextInput(
name="base_url",
display_name="NVIDIA Base URL",
refresh_button=True,
value="https://integrate.api.nvidia.com/v1",
required=True,
),
SecretStrInput(
name="nvidia_api_key",
display_name="NVIDIA API Key",
info="The NVIDIA API Key.",
advanced=False,
value="NVIDIA_API_KEY",
required=True,
),
FloatInput(
name="temperature",
display_name="Model Temperature",
value=0.1,
advanced=True,
),
]
def update_build_config(self, build_config: dotdict, field_value: Any, field_name: str | None = None):
if field_name == "base_url" and field_value:
try:
build_model = self.build_embeddings()
ids = [model.id for model in build_model.available_models]
build_config["model"]["options"] = ids
build_config["model"]["value"] = ids[0]
except Exception as e:
msg = f"Error getting model names: {e}"
raise ValueError(msg) from e
return build_config
def build_embeddings(self) -> Embeddings:
try:
from langchain_nvidia_ai_endpoints import NVIDIAEmbeddings
except ImportError as e:
msg = "Please install langchain-nvidia-ai-endpoints to use the Nvidia model."
raise ImportError(msg) from e
try:
output = NVIDIAEmbeddings(
model=self.model,
base_url=self.base_url,
temperature=self.temperature,
nvidia_api_key=self.nvidia_api_key,
)
except Exception as e:
msg = f"Could not connect to NVIDIA API. Error: {e}"
raise ValueError(msg) from e
return output
Ollama Embeddings
This component generates embeddings using Ollama models.
Use this component to create embeddings with locally run Ollama models.
Parameters
Name | Display Name | Info |
---|---|---|
Ollama Model |
Model Name |
Name of the Ollama model to use |
Ollama Base URL |
Base URL |
Base URL of the Ollama API |
Model Temperature |
Temperature |
Temperature parameter for the model |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using Ollama. |
Component code
ollama.py
from typing import Any
from urllib.parse import urljoin
import httpx
from langchain_ollama import OllamaEmbeddings
from langflow.base.models.model import LCModelComponent
from langflow.base.models.ollama_constants import OLLAMA_EMBEDDING_MODELS, URL_LIST
from langflow.field_typing import Embeddings
from langflow.io import DropdownInput, MessageTextInput, Output
HTTP_STATUS_OK = 200
class OllamaEmbeddingsComponent(LCModelComponent):
display_name: str = "Ollama Embeddings"
description: str = "Generate embeddings using Ollama models."
documentation = "https://python.langchain.com/docs/integrations/text_embedding/ollama"
icon = "Ollama"
name = "OllamaEmbeddings"
inputs = [
DropdownInput(
name="model_name",
display_name="Ollama Model",
value="",
options=[],
real_time_refresh=True,
refresh_button=True,
combobox=True,
required=True,
),
MessageTextInput(
name="base_url",
display_name="Ollama Base URL",
value="",
required=True,
),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
try:
output = OllamaEmbeddings(model=self.model_name, base_url=self.base_url)
except Exception as e:
msg = (
"Unable to connect to the Ollama API. ",
"Please verify the base URL, ensure the relevant Ollama model is pulled, and try again.",
)
raise ValueError(msg) from e
return output
async def update_build_config(self, build_config: dict, field_value: Any, field_name: str | None = None):
if field_name in {"base_url", "model_name"} and not await self.is_valid_ollama_url(field_value):
# Check if any URL in the list is valid
valid_url = ""
for url in URL_LIST:
if await self.is_valid_ollama_url(url):
valid_url = url
break
build_config["base_url"]["value"] = valid_url
if field_name in {"model_name", "base_url", "tool_model_enabled"}:
if await self.is_valid_ollama_url(self.base_url):
build_config["model_name"]["options"] = await self.get_model(self.base_url)
elif await self.is_valid_ollama_url(build_config["base_url"].get("value", "")):
build_config["model_name"]["options"] = await self.get_model(build_config["base_url"].get("value", ""))
else:
build_config["model_name"]["options"] = []
return build_config
async def get_model(self, base_url_value: str) -> list[str]:
"""Get the model names from Ollama."""
model_ids = []
try:
url = urljoin(base_url_value, "/api/tags")
async with httpx.AsyncClient() as client:
response = await client.get(url)
response.raise_for_status()
data = response.json()
model_ids = [model["name"] for model in data.get("models", [])]
# this to ensure that not embedding models are included.
# not even the base models since models can have 1b 2b etc
# handles cases when embeddings models have tags like :latest - etc.
model_ids = [
model
for model in model_ids
if any(model.startswith(f"{embedding_model}") for embedding_model in OLLAMA_EMBEDDING_MODELS)
]
except (ImportError, ValueError, httpx.RequestError) as e:
msg = "Could not get model names from Ollama."
raise ValueError(msg) from e
return model_ids
async def is_valid_ollama_url(self, url: str) -> bool:
try:
async with httpx.AsyncClient() as client:
return (await client.get(f"{url}/api/tags")).status_code == HTTP_STATUS_OK
except httpx.RequestError:
return False
OpenAI Embeddings
This component loads embedding models from OpenAI.
Use this component to generate embeddings using OpenAI’s models.
Ensure you have a valid OpenAI API key and sufficient quota.
Parameters
Name | Display Name | Info |
---|---|---|
OpenAI API Key |
API Key |
The API key to use for accessing the OpenAI API |
Default Headers |
Default Headers |
Default headers for the HTTP requests |
Default Query |
Default Query |
Default query parameters for the HTTP requests |
Allowed Special |
Allowed Special Tokens |
Special tokens allowed for processing |
Disallowed Special |
Disallowed Special Tokens |
Special tokens disallowed for processing |
Chunk Size |
Chunk Size |
Chunk size for processing |
Client |
HTTP Client |
HTTP client for making requests |
Deployment |
Deployment |
Deployment name for the model |
Embedding Context Length |
Context Length |
Length of embedding context |
Max Retries |
Max Retries |
Maximum number of retries for failed requests |
Model |
Model Name |
Name of the model to use |
Model Kwargs |
Model Arguments |
Additional keyword arguments for the model |
OpenAI API Base |
API Base URL |
Base URL of the OpenAI API |
OpenAI API Type |
API Type |
Type of the OpenAI API |
OpenAI API Version |
API Version |
Version of the OpenAI API |
OpenAI Organization |
Organization |
Organization associated with the API key |
OpenAI Proxy |
Proxy |
Proxy server for the requests |
Request Timeout |
Request Timeout |
Timeout for the HTTP requests |
Show Progress Bar |
Show Progress |
Whether to show a progress bar for processing |
Skip Empty |
Skip Empty |
Whether to skip empty inputs |
TikToken Enable |
Enable TikToken |
Whether to enable TikToken |
TikToken Model Name |
TikToken Model |
Name of the TikToken model |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using OpenAI. |
Component code
openai.py
from langchain_openai import OpenAIEmbeddings
from langflow.base.embeddings.model import LCEmbeddingsModel
from langflow.base.models.openai_constants import OPENAI_EMBEDDING_MODEL_NAMES
from langflow.field_typing import Embeddings
from langflow.io import BoolInput, DictInput, DropdownInput, FloatInput, IntInput, MessageTextInput, SecretStrInput
class OpenAIEmbeddingsComponent(LCEmbeddingsModel):
display_name = "OpenAI Embeddings"
description = "Generate embeddings using OpenAI models."
icon = "OpenAI"
name = "OpenAIEmbeddings"
inputs = [
DictInput(
name="default_headers",
display_name="Default Headers",
advanced=True,
info="Default headers to use for the API request.",
),
DictInput(
name="default_query",
display_name="Default Query",
advanced=True,
info="Default query parameters to use for the API request.",
),
IntInput(name="chunk_size", display_name="Chunk Size", advanced=True, value=1000),
MessageTextInput(name="client", display_name="Client", advanced=True),
MessageTextInput(name="deployment", display_name="Deployment", advanced=True),
IntInput(name="embedding_ctx_length", display_name="Embedding Context Length", advanced=True, value=1536),
IntInput(name="max_retries", display_name="Max Retries", value=3, advanced=True),
DropdownInput(
name="model",
display_name="Model",
advanced=False,
options=OPENAI_EMBEDDING_MODEL_NAMES,
value="text-embedding-3-small",
),
DictInput(name="model_kwargs", display_name="Model Kwargs", advanced=True),
SecretStrInput(name="openai_api_key", display_name="OpenAI API Key", value="OPENAI_API_KEY", required=True),
MessageTextInput(name="openai_api_base", display_name="OpenAI API Base", advanced=True),
MessageTextInput(name="openai_api_type", display_name="OpenAI API Type", advanced=True),
MessageTextInput(name="openai_api_version", display_name="OpenAI API Version", advanced=True),
MessageTextInput(
name="openai_organization",
display_name="OpenAI Organization",
advanced=True,
),
MessageTextInput(name="openai_proxy", display_name="OpenAI Proxy", advanced=True),
FloatInput(name="request_timeout", display_name="Request Timeout", advanced=True),
BoolInput(name="show_progress_bar", display_name="Show Progress Bar", advanced=True),
BoolInput(name="skip_empty", display_name="Skip Empty", advanced=True),
MessageTextInput(
name="tiktoken_model_name",
display_name="TikToken Model Name",
advanced=True,
),
BoolInput(
name="tiktoken_enable",
display_name="TikToken Enable",
advanced=True,
value=True,
info="If False, you must have transformers installed.",
),
IntInput(
name="dimensions",
display_name="Dimensions",
info="The number of dimensions the resulting output embeddings should have. "
"Only supported by certain models.",
advanced=True,
),
]
def build_embeddings(self) -> Embeddings:
return OpenAIEmbeddings(
client=self.client or None,
model=self.model,
dimensions=self.dimensions or None,
deployment=self.deployment or None,
api_version=self.openai_api_version or None,
base_url=self.openai_api_base or None,
openai_api_type=self.openai_api_type or None,
openai_proxy=self.openai_proxy or None,
embedding_ctx_length=self.embedding_ctx_length,
api_key=self.openai_api_key or None,
organization=self.openai_organization or None,
allowed_special="all",
disallowed_special="all",
chunk_size=self.chunk_size,
max_retries=self.max_retries,
timeout=self.request_timeout or None,
tiktoken_enabled=self.tiktoken_enable,
tiktoken_model_name=self.tiktoken_model_name or None,
show_progress_bar=self.show_progress_bar,
model_kwargs=self.model_kwargs,
skip_empty=self.skip_empty,
default_headers=self.default_headers or None,
default_query=self.default_query or None,
)
Text embedder
This component generates embeddings for a given message using a specified embedding model.
Parameters
Name | Display Name | Info |
---|---|---|
embedding_model |
Embedding Model |
The embedding model to use for generating embeddings. |
message |
Message |
The message for which to generate embeddings. |
Name | Display Name | Info |
---|---|---|
embeddings |
Embedding Data |
Data object containing the original text and its embedding vector. |
Component code
text_embedder.py
import logging
from typing import TYPE_CHECKING
from langflow.custom import Component
from langflow.io import HandleInput, MessageInput, Output
from langflow.schema import Data
if TYPE_CHECKING:
from langflow.field_typing import Embeddings
from langflow.schema.message import Message
class TextEmbedderComponent(Component):
display_name: str = "Text Embedder"
description: str = "Generate embeddings for a given message using the specified embedding model."
icon = "binary"
inputs = [
HandleInput(
name="embedding_model",
display_name="Embedding Model",
info="The embedding model to use for generating embeddings.",
input_types=["Embeddings"],
required=True,
),
MessageInput(
name="message",
display_name="Message",
info="The message to generate embeddings for.",
required=True,
),
]
outputs = [
Output(display_name="Embedding Data", name="embeddings", method="generate_embeddings"),
]
def generate_embeddings(self) -> Data:
try:
embedding_model: Embeddings = self.embedding_model
message: Message = self.message
# Combine validation checks to reduce nesting
if not embedding_model or not hasattr(embedding_model, "embed_documents"):
msg = "Invalid or incompatible embedding model"
raise ValueError(msg)
text_content = message.text if message and message.text else ""
if not text_content:
msg = "No text content found in message"
raise ValueError(msg)
embeddings = embedding_model.embed_documents([text_content])
if not embeddings or not isinstance(embeddings, list):
msg = "Invalid embeddings generated"
raise ValueError(msg)
embedding_vector = embeddings[0]
self.status = {"text": text_content, "embeddings": embedding_vector}
return Data(data={"text": text_content, "embeddings": embedding_vector})
except Exception as e:
logging.exception("Error generating embeddings")
error_data = Data(data={"text": "", "embeddings": [], "error": str(e)})
self.status = {"error": str(e)}
return error_data
VertexAI Embeddings
This component wraps around Google Vertex AI Embeddings API.
Use this component to generate embeddings using Google’s Vertex AI service.
Ensure you have the necessary Google Cloud credentials and permissions.
Parameters
Name | Display Name | Info |
---|---|---|
credentials |
Credentials |
The default custom credentials to use |
location |
Location |
The default location to use when making API calls |
max_output_tokens |
Max Output Tokens |
Token limit for text output from one prompt |
model_name |
Model Name |
The name of the Vertex AI large language model |
project |
Project |
The default GCP project to use when making Vertex API calls |
request_parallelism |
Request Parallelism |
The amount of parallelism allowed for requests |
temperature |
Temperature |
Tunes the degree of randomness in text generations |
top_k |
Top K |
How the model selects tokens for output |
top_p |
Top P |
Probability threshold for token selection |
tuned_model_name |
Tuned Model Name |
The name of a tuned model (overrides model_name if provided) |
verbose |
Verbose |
Controls the level of detail in the output |
Name | Type | Description |
---|---|---|
embeddings |
Embeddings |
An instance for generating embeddings using VertexAI. |
Component code
vertexai.py
from langflow.base.models.model import LCModelComponent
from langflow.field_typing import Embeddings
from langflow.io import BoolInput, FileInput, FloatInput, IntInput, MessageTextInput, Output
class VertexAIEmbeddingsComponent(LCModelComponent):
display_name = "VertexAI Embeddings"
description = "Generate embeddings using Google Cloud VertexAI models."
icon = "VertexAI"
name = "VertexAIEmbeddings"
inputs = [
FileInput(
name="credentials",
display_name="Credentials",
info="JSON credentials file. Leave empty to fallback to environment variables",
value="",
file_types=["json"],
required=True,
),
MessageTextInput(name="location", display_name="Location", value="us-central1", advanced=True),
MessageTextInput(name="project", display_name="Project", info="The project ID.", advanced=True),
IntInput(name="max_output_tokens", display_name="Max Output Tokens", advanced=True),
IntInput(name="max_retries", display_name="Max Retries", value=1, advanced=True),
MessageTextInput(name="model_name", display_name="Model Name", value="textembedding-gecko", required=True),
IntInput(name="n", display_name="N", value=1, advanced=True),
IntInput(name="request_parallelism", value=5, display_name="Request Parallelism", advanced=True),
MessageTextInput(name="stop_sequences", display_name="Stop", advanced=True, is_list=True),
BoolInput(name="streaming", display_name="Streaming", value=False, advanced=True),
FloatInput(name="temperature", value=0.0, display_name="Temperature"),
IntInput(name="top_k", display_name="Top K", advanced=True),
FloatInput(name="top_p", display_name="Top P", value=0.95, advanced=True),
]
outputs = [
Output(display_name="Embeddings", name="embeddings", method="build_embeddings"),
]
def build_embeddings(self) -> Embeddings:
try:
from langchain_google_vertexai import VertexAIEmbeddings
except ImportError as e:
msg = "Please install the langchain-google-vertexai package to use the VertexAIEmbeddings component."
raise ImportError(msg) from e
from google.oauth2 import service_account
if self.credentials:
gcloud_credentials = service_account.Credentials.from_service_account_file(self.credentials)
else:
# will fallback to environment variable or inferred from gcloud CLI
gcloud_credentials = None
return VertexAIEmbeddings(
credentials=gcloud_credentials,
location=self.location,
max_output_tokens=self.max_output_tokens or None,
max_retries=self.max_retries,
model_name=self.model_name,
n=self.n,
project=self.project,
request_parallelism=self.request_parallelism,
stop=self.stop_sequences or None,
streaming=self.streaming,
temperature=self.temperature,
top_k=self.top_k or None,
top_p=self.top_p,
)