Develop applications with Spring and Astra DB Serverless
Spring is a popular framework for building Java applications.
Spring Boot is a project within the Spring ecosystem that simplifies the configuration of Spring applications through convenient dependency descriptors called starters
.
Spring Data for Apache Cassandra® (spring-data-cassandra
) is a Spring Data project that provides easy configuration and access to databases that are based on Apache Cassandra®, including Astra DB databases.
While you can use Spring Data for Apache Cassandra (spring-data-cassandra
) without Spring Boot, Spring applications commonly use Spring Data projects through Spring Boot starters, like spring-boot-starter-data-cassandra
(associated with the standard web stack) or spring-boot-starter-data-cassandra-reactive
(named for the reactive stack).
spring-data-cassandra
uses the Apache Cassandra Java driver to connect to your database, and it provides abstractions with Spring concepts like templates, repositories, and entities.
The stateful object CqlSession
is instantiated and injected into Spring’s CassandraTemplate
, also known as CassandraOperations
.
You can use CqlSession
directly from CassandraTemplate
or you can inject CqlSession
into a different CassandraRepository
, which is a specialization of Spring Data’s CrudRepository
for Apache Cassandra.
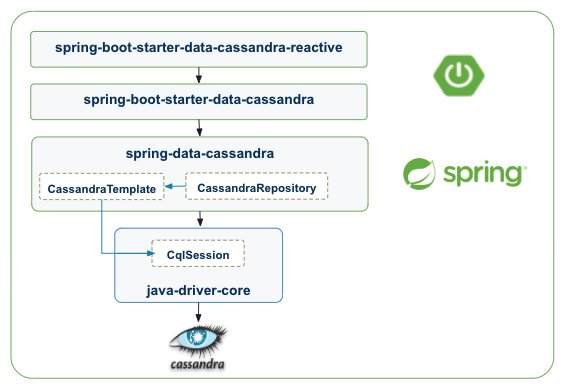
This guide demonstrates how to connect to Astra with spring-boot-starter-data-cassandra
.
Prerequisites
This integration requires familiarity with Spring and Java.
You need Spring Boot version 2.1 or later and compatible versions of Spring Framework, Java/JDK, and Maven. For more information, see Spring Boot supported versions and Spring Boot System Requirements.
This guide uses Maven as an example. If you prefer to use Gradle, you must modify the commands and configurations accordingly.
If you prefer to use Spring Data without Spring Boot, you need Spring Data version 3.0.0 or later.
DataStax recommends using the latest versions whenever possible.
Prepare Astra DB
-
Create an Astra DB database or use an existing one.
-
Generate an application token with the Database Administrator role.
-
Download your database’s Secure Connect Bundle (SCB).
You project requires access to this zip file to connect to your database. For this demo, you can store the file relative to the directory where you plan to build and run the demo project. In a production scenario, treat the SCB as you would any other sensitive credentials.
Set up the demo project
This guide uses a sample to-do list app to demonstrate how you can use spring-boot-starter-data-cassandra
to connect to your Astra DB database.
Connectivity to Astra is handled by the Apache Cassandra Java driver through abstractions in Spring Data for Apache Cassandra. For more information, see Connecting to Cassandra with Spring.
-
Clone the
sample to-do list app repo
. -
Open
/src/main/resources/application.properties
, and then edit the configuration as needed:spring.cassandra.username=token spring.cassandra.keyspace-name=KEYSPACE_NAME spring.cassandra.schema-action=CREATE_IF_NOT_EXISTS spring.cassandra.request.timeout=10s spring.cassandra.connection.connect-timeout=10s spring.cassandra.connection.init-query-timeout=10s spring.cassandra.password=APPLICATION_TOKEN datastax.astra.secure-connect-bundle=PATH_TO_SCB
Replace the following:
-
KEYSPACE_NAME
: The name of the keyspace in your database that you want you application to connect to. The default value isdefault_keyspace
. If you are using an existing database that already contains data, consider creating a keyspace for this demo. -
APPLICATION_TOKEN
: An application token with the Database Administrator role. -
PATH_TO_SCB
: The path to your database’s SCB zip file.
-
-
Recommended: Specify the latest version of the Apache Cassandra Java driver
in the demo project’s
pom.xml
:-
Latest version
-
Version 4.17 and earlier
<dependency> <groupId>org.apache.cassandra</groupId> <artifactId>java-driver-core</artifactId> <version>VERSION</version> </dependency>
<dependency> <groupId>com.datastax.oss</groupId> <artifactId>java-driver-core</artifactId> <version>VERSION</version> </dependency>
Although
spring-data-cassandra
includes a version of the Java driver, DataStax recommends specifying the latest version. For this demo, the minimum supported version is 4.6.0.If you choose to install an earlier version, make sure you choose a version that is compatible with Astra DB. If you need to query vector data, make sure your chosen version also supports vector data. For more information, see Cassandra drivers supported by DataStax.
-
-
Build and run the application:
mvn spring-boot:run
At startup, the application creates a table in your database. This can take a few minutes.
You can ping the app to check that it is running:
curl http://localhost:8080
Try the demo project
The demo project is a simple to-do list application that stores the to-do list items in a table. You can use these command to interact with the demo app:
-
Fetch the list:
curl -X GET http://localhost:8080/todos
Initially, the table is empty. After you add a task, this command returns the contents of the to-do list table.
-
Add a task to the list:
curl -X POST http://localhost:8080/todos \ -H "Content-Type: application/json" \ -d '{"title": "TASK_NAME", "description": "TASK_DESCRIPTION", "completed": false}'
If you want to compare your requests with the raw table data, you can use cqlsh
to query the table directly:
select * from KEYSPACE_NAME.todos;
Replace KEYSPACE_NAME
with the keyspace you specified in application.properties
.
Spring Data Cassandra best practices
- Define your own
CqlSession
bean and letSpring-Data
find it -
The Spring Data Cassandra starters provide some dedicated keys in the configuration file,
application.properties
. These keys are prefixed byspring.data.cassandra.*
. However, this is not an exhaustive list of driver options.Similarly, some super classes, like
AbstractCassandraConfiguration
, are provided in such a way that you can specify a few configuration properties with a limited set of keys. - Don’t use
findAll()
-
This demo uses
findAll()
for simplicity. However, this can be a resource-intensive and long-running operation on tables of any significant size.This method skips the default paging mechanism and retrieves all records in the table. Effectively, it performs a full scan of the cluster, selecting data for each node. Given that Cassandra tables are designed to store billions of records, this can be extremely slow and cause
OutOfMemoryException
.Before adapting this demo for your own projects, replace
findAll()
withfind()
. - Don’t use
@AllowFiltering
-
The
@AllowFiltering
annotation corresponds to the CQL statementALLOW FILTERING
.Only use this annotation in situations where you provide the partition key and you know your partition size is relatively small.
Most of the time, using
@AllowFiltering
indicates a problem with your data model, such as a poorly designed primary key. You might need to create another table to store the same data or create a secondary index. - Don’t rely exclusively on Spring Data to create your schema
-
This demo uses
spring.data.cassandra.schema-action: CREATE_IF_NOT_EXISTS
in theapplication.properties
configuration file. This configuration attempts to create tables based on your annotated beans.DataStax does not recommend using this configuration key in production scenarios because it can lead to bad data models, and it doesn’t provide access to find-grained properties like
COMPACTION
andTTL
(if supported) that can be different in development and production environments.Instead, create schemas with DDL scripts, as in
schema.cql
, and execute those intentionally to build your schemas. For more information, see Initialize a Database using Basic SQL Scripts and Spring Data Cassandra Schema Management. - Consider your data model before your entities
-
With the
JPA
(entity, repository) methodology, it can be tempting to reuse the same entities and repositories to perform multiple queries against the same table. However, most new requests won’t valid because you won’t use the primary key.In response, it can be equally tempting to create a secondary index or use
@AllowFiltering
. DataStax does not recommend this.Instead, create a separate table, entity, and repository, even if they contain the same data. Generally, there is a 1:1 relationship between queries and tables.
CassandraRepository
cannot implement everything-
In production applications, you might need to go back to the
CqlSession
and execute custom fine-grained queries, such as batches, TTL, or LWT. The Spring Data Cassandra interfaces andCassandraRepository
aren’t sufficient for these queries.Instead, you can extend the
SimpleCassandraRepository
class, which is an abstract class (not an interface) that provides direct access toCqlSession
, allowing you to execute queries as needed. For example:package com.datastax.workshop.todo; import java.util.UUID; import org.springframework.data.cassandra.core.CassandraOperations; import org.springframework.data.cassandra.core.mapping.CassandraPersistentEntity; import org.springframework.data.cassandra.repository.support.MappingCassandraEntityInformation; import org.springframework.data.cassandra.repository.support.SimpleCassandraRepository; import org.springframework.stereotype.Repository; import com.datastax.oss.driver.api.core.CqlSession; @Repository public class TodoRepositorySimpleCassandra extends SimpleCassandraRepository<TodoEntity, UUID> { protected final CqlSession cqlSession; protected final CassandraOperations cassandraTemplate; @SuppressWarnings("unchecked") public TodoRepositorySimpleCassandra(CqlSession cqlSession, CassandraOperations ops) { super(new MappingCassandraEntityInformation<TodoEntity, UUID>( (CassandraPersistentEntity<TodoEntity>) ops.getConverter().getMappingContext() .getRequiredPersistentEntity(TodoEntity.class), ops.getConverter()), ops); this.cqlSession = cqlSession; this.cassandraTemplate = ops; } }
See also
Learn more and find more examples in the Spring Data Cassandra and the Apache Cassandra Java driver documentation.
For an example of Spring Data Cassandra without Spring Boot, see the astra-spring-data-standalone
example repository.